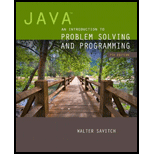
Concept explainers
Repeat Exercise 2 in Chapter 7, but use an instance of ArrayList instead of an array. Do not read the number of values, but continue to read values until the user enters a negative value.

Explanation of Solution
Program Plan:
- Import required package.
- Define “CountFamiles”class.
- Define main function.
- Create object for scanner class.
- Create array “incomeArr” by using “ArrayList”.
- Display the prompt statement.
- Assign “additionalValues” to “true”.
- Initializes required variable.
- Read input of income from user up to the value of “additionalValues” is “false”.
- Display prompt statement for each family.
- Read each family income from user and store it to “incomeValues”.
- If “incomeValues” is less than “0”, then set “additionalValues” to “false”.
- Otherwise, add the “incomeValues” to array “incomeArr”.
- Increment the index.
- Compute maximum income using “for” loop.
- If “incomeArr.get(i)” is greater than maximum income, then assign maximum income to “incomeArr.get(i)”.
- Display maximum income.
- Display “10” percent of maximum income by “0.1 * maximumIncome”.
- Initializes the count value to “0”.
- Compute the count of families with incomes less than “10” percentage of maximum income using “for” loop.
- Check condition. If value of “incomeArr.get(i)” is less than “0.1 * maximumIncome”, then increment count value and display the family income with less than “10” percentage of income by family wise.
- Finally display the count of families.
- Define main function.
Program:
The below java program is used to counts the number of families with less than “10” percent of maximum income using array.
“CountFamiles.java”
//Import required package
import java.util.*;
//Define "CountFamiles" class
public class CountFamiles
{
//Define main function
public static void main(String[] args)
{
//Create object for scanner class
Scanner input = new Scanner(System.in);
/* Create array "incomeArr" by using "ArrayList" */
ArrayList<Double> incomeArr = new ArrayList<Double>();
//Display the prompt statement
System.out.println("Enter the income for each family. Use a negative value to indicate the end ");
//Assign "additionalValues" to "true"
boolean additionalValues = true;
//Initializes required variable
int i = 0;
/* Read input of income from user upto the value of "additionalValues" is "false" */
while(additionalValues)
{
//Display prompt statement for each family
System.out.print("Enter Income for family " + (i +1) + ": ");
/* Read each family income from user and store it to "incomeValues" */
double incomeValues = input.nextDouble();
/* If "incomeValues" is less than "0", then */
if(incomeValues < 0)
/* Set "additionalValues" to "false" */
additionalValues = false;
/* Otherwise */
else
/* Add the "incomeValues" to array "incomeArr" */
incomeArr.add(incomeValues);
//Increment the index
i++;
}
//Compute maximum income using "for" loop
double maximumIncome = incomeArr.get(0);
for(i = 0; i < incomeArr.size(); i ++)
{
/* If incomeArr.get[i] is greater than maximum income, then */
if(incomeArr.get(i) > maximumIncome)
//Assign maximum income to "incomeArr.get(i)"
maximumIncome = incomeArr.get(i);
}
//Display maximum income
System.out.println("The maximum income is: " + maximumIncome);
//Display 10 percent of maximum income
System.out.println("10% of maximum income is: " + (0.1 * maximumIncome));
//Display prompt statement
System.out.println("\nDisplaying all families with incomes less than 10% of the maximum income income");
//Initializes the count value to "0"
int countValue = 0;
//Compute the count of families with incomes less than 10% of maximum income
for( i=0; i<incomeArr.size(); i++)
{
//Check condition
if(incomeArr.get(i) < (0.1 * maximumIncome))
{
//Increment count value
countValue++;
/* Display the family income with less than 10 percentage of income by family wise */
System.out.println("Family " + (i+1) + " had income " + incomeArr.get(i));
}
}
//Display count of families
System.out.println("Count of families with 10% of maximum income is: " + countValue);
}
}
Output:
Enter the income for each family. Use a negative value to indicate the end
Enter Income for family 1: 10000
Enter Income for family 2: 48000
Enter Income for family 3: 20000
Enter Income for family 4: 12000
Enter Income for family 5: 300000
Enter Income for family 6: 40000
Enter Income for family 7: -1
The maximum income is: 300000.0
10% of maximum income is: 30000.0
Displaying all families with incomes less than 10% of the maximum income
Family 1 had income 10000.0
Family 3 had income 20000.0
Family 4 had income 12000.0
Count of families with 10% of maximum income is: 3
Want to see more full solutions like this?
Chapter 12 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
Additional Engineering Textbook Solutions
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Starting out with Visual C# (4th Edition)
Database Concepts (8th Edition)
Programming in C
Starting Out with C++ from Control Structures to Objects (8th Edition)
Starting Out with Python (3rd Edition)
- 3. Create an ArrayList of strings to store the names of celebrities or athletes. Add five names to the list. Process the list with a for loop and the get() method to display the names, one name per line. Pass the list to avoid method. Inside the method, Insert another name at index 2 and remove the name at index 4. Use a foreach loop to display the arraylist again, all names on one line separated by asterisks. After the method call in main, create an iterator for the arraylist and use it to display the list one more time. See Sample Output. SAMPLE OUTPUT Here is the list Lionel Messi Drake Adele Dwayne Johnson Beyonce Here is the new list * Lionel Messi * Drake * Taylor Swift * Adele * Beyonce Using an iterator, here is the list Lionel Messi Drake Taylor Swift Adele Beyoncearrow_forwardUse the Iterator Pattern: Create a class called Exercise, and then, in its main method, create an ArrayList called stringArrayList. Add the following five strings to stringArrayList: “one”, “two”, ”three”, ”four”, ”five”. Then, use a for loop and the index of stringArrayList to print all these five strings out to the console. 2. For stringArrayList above, can you use an iterator to traverse the five strings inside, without using the index? 3. If change stringArrayList into a TreeSet, how do you traverse the five strings use an iterator? Implement an Iterator: 4. Create a class called StringArray that is able to store a number of String objects. Create an instance variable, String[] values, (internal data storage) of StringArray to store all the strings. 5. Create a constructor for StringArray, which is able to build an object of StringArray using the parameter. public StringArray(String[] values) 6. Make the class StringArray implements Iterable So that it…arrow_forwardCreate a class containing the main method. In the main method, create an integer array and initialize it with the numbers: 1,3,5,7,9,11,13,15,17,19 Pass the array as an argument to a method. Use a loop to add 1 to each element of the array and return the array to the main method. In the main method, use a loop to add the array elements and display the result. Note: In the loops, use the array field that holds the length of the array and do not use a number for array length.arrow_forward
- Java Create an ArrayList of strings to store the names of celebrities or athletes. Add five names to the list. Process the list with a for loop and the get() method to display the names, one name per line. Pass the list to a void method. Inside the method, Insert another name at index 2 and remove the name at index 4. Use a foreach loop to display the arraylist again, all names on one line separated by asterisks. After the method call in main, create an iterator for the arraylist and use it to display the list one more time. See Sample Output. SAMPLE OUTPUT Here is the list Lionel Messi Drake Adele Dwayne Johnson Beyonce Here is the new list * Lionel Messi * Drake * Taylor Swift * Adele * Beyonce Using an iterator, here is the list Lionel Messi Drake Taylor Swift Adele Beyoncearrow_forwardWrite a program that do the following: Ask the user to input his/her first name and store it in a String variable. Create an ArrayList with the type String. Each letter of the inputted name should be stored in a separate element of the ArrayList. Print the ArrayListsarrow_forwardImplement one of the sorts described in Chapters 15 and 16. Input is an array with at least 10 items. The items in the array can be of several types (Suggested types are integers-denoting how many customers visited the store in the last three weeks or strings-denoting the names of featured items). The items should be connected to a store in some way. Use some of the given data fields Print a title indicating what the data represents. Print the original data, AND the sorted data.arrow_forward
- need Urgent Requirement: There will one question related to ArrayList class similar to the following question. The following driver has created a an ArrayList of Point objects. write a method that moves all the points with the value of x greater than its value of y to the end of the list. .arrow_forwardRename the show method to people Override the show method in student and in the other class you created (this will be the polymorphic method) In main program, create an array of 3 objects of class people Instantiate each array position with a different object (people, student, other) With a loop, display all the objects in the array.arrow_forwardA personal phone directory contains room for first names and phone numbers for 30 people. Assign names and phone numbers for the first 10 people. Prompt the user for a name, and if the name is found in the list, display the corresponding phone number. If the name is not found in the list, prompt the user for a phone number, and add the new name and phone number to the list. Continue to prompt the user for names until the user enters quit. After the arrays are full (containing 30 names), do not allow the user to add new entries.arrow_forward
- A personal phone directory contains room for first names and phone numbers for 30 people. Assign names and phone numbers for the first 10 people. Prompt the user for a name, and if the name is found in the list, display the corresponding phone number. If the name is not found in the list, prompt the user for a phone number, and add the new name and phone number to the list. Continue to prompt the user for names until the user enters quit. After the arrays are full (containing 30 names), do not allow the user to add new entries. Use the following names and phone numbers: Name Phone # Gina (847) 341-0912 Marcia (847) 341-2392 Rita (847) 354-0654 Jennifer (414) 234-0912 Fred (414) 435-6567 Neil (608) 123-0904 Judy (608) 435-0434 Arlene (608) 123-0312 LaWanda (920) 787-9813 Deepak (930) 412-0991arrow_forwardA personal phone directory contains room for first names and phone numbers for 30 people. Assign names and phone numbers for the first 10 people. Prompt the user for a name, and if the name is found in the list, display the corresponding phone number. If the name is not found in the list, prompt the user for a phone number, and add the new name and phone number to the list. Continue to prompt the user for names until the user enters quit. After the arrays are full (containing 30 names), do not allow the user to add new entries. Using Scanner.input to prompt user, Use the following names and phone numbers: Gina (847) 341-0912 Marcia (847) 341-2392 Rita (847) 354-0654 Jennifer (414) 234-0912 Fred (414) 435-6567 Neil (608) 123-0904 Judy (608) 435-0434 Arlene (608) 123-0312 LaWanda (920) 787-9813 Deepak (930) 412-0991arrow_forwardA personal phone directory contains room for first names and phone numbers for 30 people. Assign names and phone numbers for the first 10 people. Prompt the user for a name, and if the name is found in the list, display the corresponding phone number. If the name is not found in the list, prompt the user for a phone number, and add the new name and phone number to the list. Continue to prompt the user for names until the user enters quit. After the arrays are full (containing 30 names), do not allow the user to add new entries. Use the following names and phone numbers: Name Phone # Gina (847) 341-0912 Marcia (847) 341-2392 Rita (847) 354-0654 Jennifer (414) 234-0912 Fred (414) 435-6567 Neil (608) 123-0904 Judy (608) 435-0434 Arlene (608) 123-0312 LaWanda (920) 787-9813 Deepak (930) 412-0991 This is code that was given to us; import java.util.*; class PhoneNumbers { public static void main(String[] args) { // Write your code here } }arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
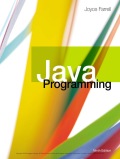