AI_Project2
.pdf
keyboard_arrow_up
School
University of North Texas *
*We aren’t endorsed by this school
Course
5300
Subject
Computer Science
Date
Dec 6, 2023
Type
Pages
8
Uploaded by amulyam232 on coursehero.com
Link -
AI Project2.ipynb - Colaboratory (google.com)
R1
import
networkx
as
nx
#import
networkx, download if not available
G = nx.DiGraph()
#code for the
network graph given in tutorial 4
G.add_edges_from([
(
0
,
1
, {
'flow'
:
2
,
'capacity'
:
6
}),
(
0
,
2
, {
'flow'
:
3
,
'capacity'
:
3
}),
(
0
,
3
, {
'flow'
:
4
,
'capacity'
:
5
}),
(
1
,
2
, {
'flow'
:
1
,
'capacity'
:
5
}),
(
1
,
5
, {
'flow'
:
1
,
'capacity'
:
3
}),
(
2
,
4
, {
'flow'
:
2
,
'capacity'
:
9
}),
(
2
,
5
, {
'flow'
:
4
,
'capacity'
:
8
}),
(
3
,
2
, {
'flow'
:
2
,
'capacity'
:
2
}),
(
3
,
4
, {
'flow'
:
2
,
'capacity'
:
3
}),
(
4
,
5
, {
'flow'
:
4
,
'capacity'
:
5
})
])
def
heuristic
(G, path):
# calculate heuristic value based on
the tutorial3
min_capacity_diff =
float
(
'inf'
)
for
u, v
in
path:
edge_data = G[u][v]
flow = edge_data.get(
'flow'
,
0
)
capacity = edge_data.get(
'capacity'
,
float
(
'inf'
))
#to know how much flow can be increased
capacity_diff = (capacity - flow)
min_capacity_diff =
min
(min_capacity_diff, capacity_diff)
return
(min_capacity_diff)
def
optimal_path
(G, source, target):
#function to find
optimal path from source to sink
paths =
list
(nx.all_simple_edge_paths(G,
source
=source,
target
=target,
cutoff
=
9
))
if not
paths:
return None
max_heuristic_value = -
1
#condition
when there exists no optimal flow
best_path =
None
for
path
in
paths:
#looping to check each path based on heuristic and return the best path
heuristic_value = (heuristic(G, path))
if
heuristic_value > max_heuristic_value:
max_heuristic_value = heuristic_value
best_path = path
return
best_path
def
update_flow
(G, path):
# update the
flow for each path in the network after increasing the flow
min_capacity = (
min
(G[u][v][
'capacity'
] - G[u][v][
'flow'
]
for
u, v
in
path))
for
u, v
in
path:
G[u][v][
'flow'
] += min_capacity
if
G.has_edge(v, u):
G[v][u][
'capacity'
] = G[v][u].get(
'capacity'
,
0
) + min_capacity
def
successor_function
(G, source, target):
#a sucessor
function to call heuristic function
while True
:
best_path =
optimal_path(G, source, target)
if
best_path
is None or
heuristic(G, best_path) ==
0
:
#calling the heuristic funtion if there exists
break
update_flow(G, best_path)
return
G
updated_G = successor_function(G,
source
=
0
,
target
=
5
)
for
path
in
nx.all_simple_edge_paths(updated_G,
source
=
0
,
target
=
5
,
cutoff
=
9
):
updated_path = [(u, v, {
'flow'
: updated_G[u][v][
'flow'
],
'capacity'
:
updated_G[u][v][
'capacity'
]})
for
u, v
in
path]
print
(updated_path)
#
updated graph/ output
R2
import
networkx
as
nx
import
random
G = nx.gnp_random_graph(
5
,
0.6
,
directed
=
True
)
# Generate a directed graph with 30 nodes
nx.is_weakly_connected(G)
if
'S'
not in
G:
# Add 'S' and 'T' nodes
G.add_node(
'S'
)
if
'T'
not in
G:
G.add_node(
'T'
)
if not
G.has_edge(
'S'
,
'T'
):
# Ensure an edge
between 'S' and 'T' with capacities and flows
capacity = random.randint(
1
,
10
)
flow = random.randint(
1
, capacity)
G.add_edge(
'S'
,
'T'
,
capacity
=capacity,
flow
=flow)
for
u, v
in
G.edges():
# Set capacities and
flows for other edges in the graph
if
u !=
'S'
and
v !=
'T'
and not
(
'capacity'
in
G[u][v]
and
'flow'
in
G[u][v]):
capacity = random.randint(
1
,
10
)
flow = random.randint(
1
, capacity)
G[u][v][
'capacity'
] = capacity
G[u][v][
'flow'
] = flow
# print("Generated Graph with Capacities and Flows:")
#
display the generated graph with capacities and flows
# for u, v in G.edges():
#
print(f"Edge: {u} -> {v} | Capacity: {G[u][v]['capacity']} | Flow:
{G[u][v]['flow']}")
def
heuristic
(G, path):
#
heuristic value calculation function
min_capacity_diff =
float
(
'inf'
)
for
u, v
in
path:
edge_data = G[u][v]
flow = edge_data.get(
'flow'
,
0
)
capacity = edge_data.get(
'capacity'
,
float
(
'inf'
))
capacity_diff = capacity - flow
min_capacity_diff =
min
(min_capacity_diff, capacity_diff)
return
min_capacity_diff
def
find_best_path
(G, source, target):
#
function to
find the best path based on the heuristic value
paths =
list
(nx.all_simple_edge_paths(G,
source
=source,
target
=target,
cutoff
=
6
))
# Limit the cutoff value
if not
paths:
return None
max_heuristic_value = -
1
best_path =
None
for
path
in
paths:
heuristic_value = heuristic(G, path)
if
heuristic_value > max_heuristic_value:
max_heuristic_value = heuristic_value
best_path = path
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
import javax.swing.*;import java.awt.*;import java.util.LinkedList;class GraphPanel extends JPanel {private LinkedList<Integer> sensorValues;public GraphPanel(LinkedList<Integer> sensorValues) {this.sensorValues = sensorValues;}public void updateValues(LinkedList<Integer> values) {this.sensorValues = values;repaint();}@Overrideprotected void paintComponent(Graphics g) {super.paintComponent(g);int xOffset = 50;int yOffset = 50;int xSpacing = (getWidth() - 2 * xOffset) / sensorValues.size();g.setColor(Color.BLACK);g.drawLine(xOffset, getHeight() - yOffset, getWidth() - xOffset, getHeight() - yOffset); // X-axisg.drawLine(xOffset, getHeight() - yOffset, xOffset, yOffset); // Y-axisg.setColor(Color.BLUE);int prevX = xOffset;int prevY = getHeight() - yOffset - (sensorValues.get(0) * (getHeight() - 2 * yOffset) / 1000);for (int i = 1; i < sensorValues.size(); i++) {int value = sensorValues.get(i);int x = xOffset + i * xSpacing;int y = getHeight() - yOffset - (value *…
arrow_forward
7- A student has created a plot of
y(t)=t^2. He needs to show another
graph of z(t)=t^3 in the same plot.
But every time he hits the plot()
function- MATLAB generates a plot
of z(t) vs t but on a different
window. What is the error?
O
It is not possible to plot multiple
plots
O He is not using the line function
Maybe he is using polar() instead of
plot()
O He is not using the hold function
arrow_forward
Given the following definition for a LinkedList:
// LinkedList.h
class LinkedList {
public: LinkedList();
// TODO: Implement me void printEveryOther() const;
private:
struct Node {
int data;
Node* next; };
Node * head; };
// LinkedList.cpp
#include "LinkedList.h"
LinkedList::LinkedList() {
head = nullptr; }
Implement the function printEveryOther, which prints every other data value (i.e. those at the odd indices assuming 0-based indexing).
arrow_forward
People is represented in the Graph by Nodes or Vertices (the vertex should consist of name of the person)
Relationships is represented in the Graph by edges or arcs
The project should have at least two class with following methods :
addNode add vertices to graphs(New person in the Graph Net )
removeNode removes vertices to graphs(remove person from the graph Net)
addEdge adds connections or paths between vertices in graphs(make new relationship between two persons in the graph )
removeEdge removes connection or paths between vertices in graphs (remove the relationship between two persons in the graph )
contains check if a graph contains a certain value
hasEdge checks if a connection or path exists between any two vertices in a graph
The output has to display a menu of choices. Then the user can select which operation want to perform. Also, you can use the method System.exit(0); to exit the program. The menu of choices will be:
Add person into the social…
arrow_forward
6 591
9 1 75
1 3 10
8 8 5 8
G746
arrow_forward
Q9-A student has to plot a graph
of f(x)=z and g(y)=z in the same
graph, with t as a parameter. The
function he uses is
O plot3(x,y,z)
plot(x,y,z)
disp
O stem(x,y)
arrow_forward
Social Graph project is network which consists of two things : People is represented in the Graph by Nodes or Vertices (the vertex should consist of name of the person) Relationships is represented in the Graph by edges or arcsThe project should have at least two class with following methods : addNode add vertices to graphs(New person in the Graph Net )removeNode removes vertices to graphs(remove person from the graph Net)addEdge adds connections or paths between vertices in graphs(make new relationship between two persons in the graph )removeEdge removes connection or paths between vertices in graphs (remove the relationship between two persons in the graph )contains check if a graph contains a certain valuehasEdge checks if a connection or path exists between any two vertices in a graph
IN JAVA LANGUAGE PLEASE and thnak you !
arrow_forward
Social Graph project is network which consists of two things : People is represented in the Graph by Nodes or Vertices (the vertex should consist of name of the person) Relationships is represented in the Graph by edges or arcsThe project should have at least two class with following methods : addNode add vertices to graphs(New person in the Graph Net )removeNode removes vertices to graphs(remove person from the graph Net)addEdge adds connections or paths between vertices in graphs(make new relationship between two persons in the graph )removeEdge removes connection or paths between vertices in graphs (remove the relationship between two persons in the graph )contains check if a graph contains a certain valuehasEdge checks if a connection or path exists between any two vertices in a graph
IN JAVA LANGUAGE
arrow_forward
1- CREATE JAVA CODE TO BUILD A GRAPH ( import from file )?
2-PRINT THE TOPOLOGICAL ORDER CRILE
3- GIVEN A SOURCE NODE PRINT THE CONTENTS USING BREADTH FIRST AND DEPTH traversal ?
4- CHECK THE GRAPH IS CONNECTED OR NOT
5- PRINT THE ADJACENCY MATRIX FOR THE GRAPH
arrow_forward
unique please
Your task for this assignment is to identify a spanning tree in one connected undirected weighted graph using C++.
Implement a spanning tree algorithm using C++. A spanning tree is a subset of the edges of a connected undirected weighted graph that connects all the vertices together, without any cycles. The program is interactive. Graph edges with respective weights (i.e., v1 v2 w) are entered at the command line and results are displayed on the console.
Each input transaction represents an undirected edge of a connected weighted graph. The edge consists of two unequal non-negative integers in the range 0 to 9 representing graph vertices that the edge connects. Each edge has an assigned weight. The edge weight is a positive integer in the range 1 to 99. The three integers on each input transaction are separated by space. An input transaction containing the string “end-of-file” signals the end of the graph edge input. After the edge information is read, the process…
arrow_forward
TranposeGraph
import java.io.*;
import java.util.*;
// This class represents a directed graph using adjacency list
// representation
class ALGraph{
private int vertices; // No. of vertices
// Array of lists for Adjacency List Representation
private LinkedList adj[];
// Constructor
ALGraph(int vertices) {
this.vertices = vertices;
adj = new LinkedList[this.vertices];
for (int i=0; i getAdjacentList(int v) {
return adj[v];
}
//Function to add an edge into the graph
void addEdge(int v, int w) {
adj[v].add(w); // Add w to v's list.
}
// TODO Transpose graph
// If the graph includes zero vertices, return null
// Create a new ALGraph
// For every vertex, retrieve its adjacent list, make a pass over the list and rewrite each edge (u, v) to be (v, u) and add the u into the adjacent list of v
public ALGraph transpose(){…
arrow_forward
A graph file is provided below. What is the total number of fan-in and fan-out of vertex 4?
# Graph example
# Number of vertices
# Directed graph
01 10
# List of edges
03 20
13 5
02 3
21 2
24 15
34 11
LO
arrow_forward
NetworkX graph generators are used to create empty graphs, digraphs, multigraphs and multidigraphs without any nodes or edges.
O True
O False
arrow_forward
Graph Theory:
Graph theory in computer science uses a graphical matrix with nodes and edges to describe a data structure. These can be used for search trees, game theory, shortest path, and many other applications in math and computer science.
An example of an application of a graph in computer science is the data structure used to hole the moves for a checkers game program. Each move can be represented by a node. The edges connecting the nodes are determined by the rules of the game, basically how to get to the node. This is a directed graph, because a player cannot take a move back. So the flow is always in one direction towards the end of the game.
Cycles in a graph is when a node can go back to itself. This is not possible in this graph, because a move can only go to another position on the board. The only case where this would be correct is if a player were allowed to skip his turn, or move to the same spot that he is already in.
A graph is said to be connected if there is a path…
arrow_forward
JAVA:
Write a program that takes a graph representing a map between cities and use Dijkstra's Algorithm to find the shortest path given a start and an end vertex. in java
1- Please comment the codes.
arrow_forward
Q- In order to plot a graph of f(x)=z
and g(y)=z in the same graph, with t as
a parameter. The function used is
plot3(x,y,z) O
plot(x,y,z) O
surf(x,y,z) O
mesh(z) O
arrow_forward
Solve problem on 79 Project Euler. This is a Graph problem in disguise, so the key is to figure out what is node and what is an edge. In other words, what are the things that have relationships in this problem and what defines their relationships?
Once you figure that out, you can use topological sort to solve the problem. Create an account on the site to confirm your answer.
You can do this in any language, but a key to solving the problem is finding the indegree of each node. The indegree of a node in a directed graph is the number of edges that have that node as a destination,1 or the number of edges that lead into the node. JUNG has a built in method for graphs to do this.
The other thing to keep in mind for JUNG is that you can’t make two edges with the same value. So if you’re using a String to represent edges, you can’t have two edges with the value of "A".
arrow_forward
Implement Two Way Linked List
The MyLinkedList class is a one-way directional linked list that enables one-way traversal of the list. MyLinkedList.java is attached below. Modify the Node class to add the new field named previous to refer to the previous node in the list, as follows:
public class Node <E> { E element;
Node<E> next; Node<E> previous;
public Node(E e) { element = e;
} }
Implement a new class named MyTwoWayLinkedList that uses a double linked list to store elements. The MyLinkedList class in the text extends MyList. Define MyTwoWayLinkedList to extend the java.util.AbstractSequentialList class. You can find methods of AbstractSequentialList from this article: https://www.geeksforgeeks.org/abstractsequentiallist-in-java-with-examples/. Study MyLinkedList.java carefully to understand how it is structured, and where and what you need to modify/implement for MyTwoWayLinkedList.
Since MyTwoWayLinkedList extends java.util.AbstractSequentialList which implements…
arrow_forward
Weighted Graph Applications Demonstration Java Data Structures.
Figure 29.23 illustrates a weighted graph with 6 vertices and 8 weighted edges.
Simply provide:
Minimal Spanning Tree as an illustration or a textual list of edges (in our standard vertex order).
Single-Source Shortest Path route from vertex 0 to the other 5 (described as one path/route for each).
draw the two solutions and attach the illustration or describe them in text (a list of edges for the one and the vertex to vertex path the other).
You can therefore attach proper content files with dot txt, png, jpg or jpeg extensions
Be sure the final trees or path lists are clearly visible in your solution. You don't need to show the solution development or progress, just the result.
arrow_forward
Information Cascades
It’s election season and two candidates, Candidate A and Candidate B, are in a hotly contested city council race in rainy Eastmoreland. You are a strategic advisor for Candidate A in charge of election forecasting and voter acquisition tactics.
Based on careful modeling, you’ve created one possible version of the social graph of voters. Your graph has 10,000 nodes, where nodes are denoted by an integer ID between 0 and 9999. The edge lists of the graphs are provided in the homework bundle. Both graphs are undirected.
Given the hyper-partisan political climate of Eastmoreland, most voters have already made up their minds: 40% know they will vote for A, 40% know they will vote for B, and the remaining 20% are undecided. Each voter’s support is determined by the last digit of their node id. If the last digit is 0–3, the node supports A. If the last digit is 4–7, the node supports B. And if the last digit is 8 or 9, the node is undecided.
The undecided voters will go…
arrow_forward
.Define request edge and assignment edge.
arrow_forward
Write a function, called getadjacents, that receives a T item and an empty queue. The function will fill the queue with all items stored in vertices that are adjacent to the vertex containing the item sent as a parameter.
The function belongs to a graph class with an adjacency list implementation. C++
arrow_forward
In this assignment, you will design the AddNode and AddEdge methods for the supplied graph data structure. The AddNode and AddEdge methods are to support the construction of undirected (bi-directional) graphs. That is if node A is connected to node B then node B is also connected to node A.
In addition to the AddNode and AddEdge methods, create a method called BreadthFirstSearch that accepts a starting node and performs a Breadth First Search of the graph. The algorithm for the breadth first traversal is provided below
1. Add a node to the queue (starting node)
2. While the queue is not empty, dequeue a node
3. Add all unvisited nodes of the dequeued node from step 2 and add them to queue
4. End While
Demonstrate your methods by creating the graph depicted in Figure 1 below and running the Breadth First Search on the graph using 0 as the starting node.
(see image below)
You may use C++, C#, to implement this program as long as the following requirements are met. A C++, or C#…
arrow_forward
class Dijkstra(): """ A fully connected directed graph with edge weights """
def __init__(self, vertex_count): self.vertex_count = vertex_count self.graph = [[0 for _ in range(vertex_count)] for _ in range(vertex_count)]
def min_distance(self, dist, min_dist_set): """ Find the vertex that is closest to the visited set """ min_dist = float("inf") for target in range(self.vertex_count): if min_dist_set[target]: continue if dist[target] < min_dist: min_dist = dist[target] min_index = target return min_index
def dijkstra(self, src): """ Given a node, returns the shortest distance to every other node """ dist = [float("inf")] * self.vertex_count dist[src] = 0 min_dist_set = [False] * self.vertex_count
for _ in range(self.vertex_count): #minimum distance vertex that is not processed…
arrow_forward
Please fill in all the code gaps if possible: (java)
public class LinkedList {
privateLinkedListNode head;
**Creates a new instance of LinkedList**
public LinkedList () {
}
public LinkedListNode getHead()
public void setHead (LinkedListNode head)
**Add item at the front of the linked list**
public void insertFront (Object item)
**Remove item at the front of linked list and return the object variable**
public Object removeFront()
}
arrow_forward
Python Graph Algorithms: Minimum Spanning Trees
Suppose you are an engineer working on designing a road network for a new town. The town has many residential areas and commercial centers that need to be connected efficiently. You decided to represent the town as a connected, undirected graph where each vertex represents a location, and each edge represents a road connecting two locations. The weight of each edge represents the distance between the two locations.
To ensure the road network is efficient, you need to find the minimum spanning tree of the graph. However, due to budget constraints, you can only construct roads with a maximum distance limit. You need to determine for how many pairs of locations, the minimum spanning tree of the graph remains the same when the maximum distance limit of a road is increased by 2 units.
To solve this, you write a program that takes as input the number of locations, the number of roads, and the weight of each road. Your program will also receive…
arrow_forward
IN java
Make a dijkestra algorithm that is efficient with large data sets
Runs Dijkstra's algorithm on the graph, starting at the node specified by the start parameter.
* Return a map where the key is the name of the node and the value is the distance from the start node.
* The start node should be included in the returned map, with the value 0 as the distance.
*
* @param start - a5.Node to start Dijkstra's algorithm at
* @return Map of all the nodes in the graph and the distance to the start a5.Node.
*/
these is the description of the method
the method signature is below
public Map<String, Double> dijkstra(String start);
Class that this method is in is below
public class GraphImpl implements Graph {
Map<String, Node> nodes; //do not delete, use this field to store your nodes
// key: name of node. value: a5.Node object associated with name
privateinttotaledges;
publicGraphImpl() {
nodes = newHashMap<>();…
arrow_forward
Java -
It is preferable to use an edge list for small graph problems because of the simplified methods provided for the linked list class definitions. True or False?
arrow_forward
Starter code for ShoppingList.java
import java.util.*;import java.util.LinkedList;
public class ShoppingList{ public static void main(String[] args) { Scanner scnr=new Scanner(System.in); LinkedList<ListItem>shoppingList=new LinkedList<ListItem>();//declare LinkedList String item; int i=0,n=0;//declare variables item=scnr.nextLine();//get input from user while(item.equals("-1")!=true)//get inputuntil user not enter -1 { shoppingList.add(new ListItem(item));//add into shoppingList LinkedList n++;//increment n item=scnr.nextLine();//get item from user } for(i=0;i<n;i++) { shoppingList.get(i).printNodeData();//call printNodeData()for each object } }}
class ListItem{ String item; //constructor ListItem(String item) { this.item=item; } void printNodeData() { System.out.println(item); }}
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
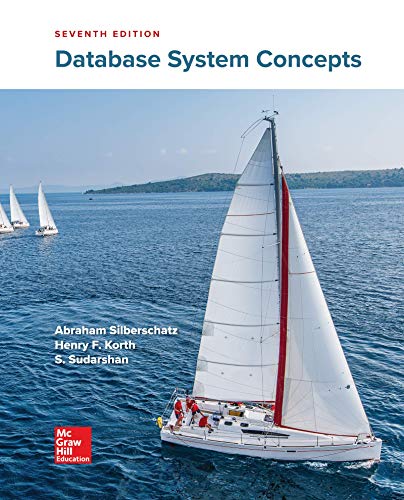
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
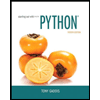
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
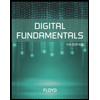
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
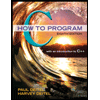
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
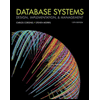
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
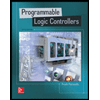
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- import javax.swing.*;import java.awt.*;import java.util.LinkedList;class GraphPanel extends JPanel {private LinkedList<Integer> sensorValues;public GraphPanel(LinkedList<Integer> sensorValues) {this.sensorValues = sensorValues;}public void updateValues(LinkedList<Integer> values) {this.sensorValues = values;repaint();}@Overrideprotected void paintComponent(Graphics g) {super.paintComponent(g);int xOffset = 50;int yOffset = 50;int xSpacing = (getWidth() - 2 * xOffset) / sensorValues.size();g.setColor(Color.BLACK);g.drawLine(xOffset, getHeight() - yOffset, getWidth() - xOffset, getHeight() - yOffset); // X-axisg.drawLine(xOffset, getHeight() - yOffset, xOffset, yOffset); // Y-axisg.setColor(Color.BLUE);int prevX = xOffset;int prevY = getHeight() - yOffset - (sensorValues.get(0) * (getHeight() - 2 * yOffset) / 1000);for (int i = 1; i < sensorValues.size(); i++) {int value = sensorValues.get(i);int x = xOffset + i * xSpacing;int y = getHeight() - yOffset - (value *…arrow_forward7- A student has created a plot of y(t)=t^2. He needs to show another graph of z(t)=t^3 in the same plot. But every time he hits the plot() function- MATLAB generates a plot of z(t) vs t but on a different window. What is the error? O It is not possible to plot multiple plots O He is not using the line function Maybe he is using polar() instead of plot() O He is not using the hold functionarrow_forwardGiven the following definition for a LinkedList: // LinkedList.h class LinkedList { public: LinkedList(); // TODO: Implement me void printEveryOther() const; private: struct Node { int data; Node* next; }; Node * head; }; // LinkedList.cpp #include "LinkedList.h" LinkedList::LinkedList() { head = nullptr; } Implement the function printEveryOther, which prints every other data value (i.e. those at the odd indices assuming 0-based indexing).arrow_forward
- People is represented in the Graph by Nodes or Vertices (the vertex should consist of name of the person) Relationships is represented in the Graph by edges or arcs The project should have at least two class with following methods : addNode add vertices to graphs(New person in the Graph Net ) removeNode removes vertices to graphs(remove person from the graph Net) addEdge adds connections or paths between vertices in graphs(make new relationship between two persons in the graph ) removeEdge removes connection or paths between vertices in graphs (remove the relationship between two persons in the graph ) contains check if a graph contains a certain value hasEdge checks if a connection or path exists between any two vertices in a graph The output has to display a menu of choices. Then the user can select which operation want to perform. Also, you can use the method System.exit(0); to exit the program. The menu of choices will be: Add person into the social…arrow_forward6 591 9 1 75 1 3 10 8 8 5 8 G746arrow_forwardQ9-A student has to plot a graph of f(x)=z and g(y)=z in the same graph, with t as a parameter. The function he uses is O plot3(x,y,z) plot(x,y,z) disp O stem(x,y)arrow_forward
- Social Graph project is network which consists of two things : People is represented in the Graph by Nodes or Vertices (the vertex should consist of name of the person) Relationships is represented in the Graph by edges or arcsThe project should have at least two class with following methods : addNode add vertices to graphs(New person in the Graph Net )removeNode removes vertices to graphs(remove person from the graph Net)addEdge adds connections or paths between vertices in graphs(make new relationship between two persons in the graph )removeEdge removes connection or paths between vertices in graphs (remove the relationship between two persons in the graph )contains check if a graph contains a certain valuehasEdge checks if a connection or path exists between any two vertices in a graph IN JAVA LANGUAGE PLEASE and thnak you !arrow_forwardSocial Graph project is network which consists of two things : People is represented in the Graph by Nodes or Vertices (the vertex should consist of name of the person) Relationships is represented in the Graph by edges or arcsThe project should have at least two class with following methods : addNode add vertices to graphs(New person in the Graph Net )removeNode removes vertices to graphs(remove person from the graph Net)addEdge adds connections or paths between vertices in graphs(make new relationship between two persons in the graph )removeEdge removes connection or paths between vertices in graphs (remove the relationship between two persons in the graph )contains check if a graph contains a certain valuehasEdge checks if a connection or path exists between any two vertices in a graph IN JAVA LANGUAGEarrow_forward1- CREATE JAVA CODE TO BUILD A GRAPH ( import from file )? 2-PRINT THE TOPOLOGICAL ORDER CRILE 3- GIVEN A SOURCE NODE PRINT THE CONTENTS USING BREADTH FIRST AND DEPTH traversal ? 4- CHECK THE GRAPH IS CONNECTED OR NOT 5- PRINT THE ADJACENCY MATRIX FOR THE GRAPHarrow_forward
- unique please Your task for this assignment is to identify a spanning tree in one connected undirected weighted graph using C++. Implement a spanning tree algorithm using C++. A spanning tree is a subset of the edges of a connected undirected weighted graph that connects all the vertices together, without any cycles. The program is interactive. Graph edges with respective weights (i.e., v1 v2 w) are entered at the command line and results are displayed on the console. Each input transaction represents an undirected edge of a connected weighted graph. The edge consists of two unequal non-negative integers in the range 0 to 9 representing graph vertices that the edge connects. Each edge has an assigned weight. The edge weight is a positive integer in the range 1 to 99. The three integers on each input transaction are separated by space. An input transaction containing the string “end-of-file” signals the end of the graph edge input. After the edge information is read, the process…arrow_forwardTranposeGraph import java.io.*; import java.util.*; // This class represents a directed graph using adjacency list // representation class ALGraph{ private int vertices; // No. of vertices // Array of lists for Adjacency List Representation private LinkedList adj[]; // Constructor ALGraph(int vertices) { this.vertices = vertices; adj = new LinkedList[this.vertices]; for (int i=0; i getAdjacentList(int v) { return adj[v]; } //Function to add an edge into the graph void addEdge(int v, int w) { adj[v].add(w); // Add w to v's list. } // TODO Transpose graph // If the graph includes zero vertices, return null // Create a new ALGraph // For every vertex, retrieve its adjacent list, make a pass over the list and rewrite each edge (u, v) to be (v, u) and add the u into the adjacent list of v public ALGraph transpose(){…arrow_forwardA graph file is provided below. What is the total number of fan-in and fan-out of vertex 4? # Graph example # Number of vertices # Directed graph 01 10 # List of edges 03 20 13 5 02 3 21 2 24 15 34 11 LOarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
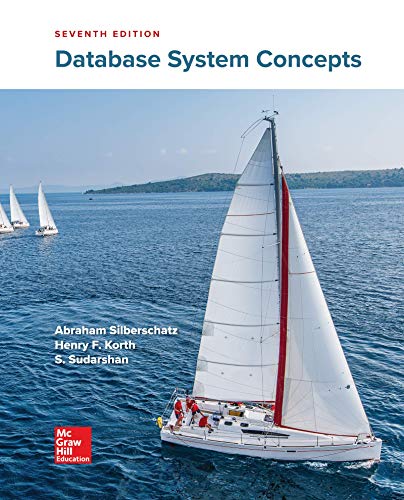
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
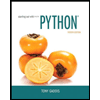
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
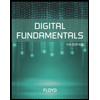
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
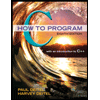
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
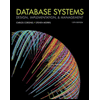
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
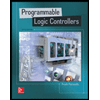
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education